1. Spring Cloud Config 개요
Spring Cloud Config는 애플리케이션의 설정을 중앙에서 관리하고 동적으로 변경할 수 있도록 도와주는 설정 서버입니다.
구성 요소:
- Config Server: 설정을 저장하고 제공하는 서버 (Git, 파일 시스템 등에서 설정을 가져옴)
- Config Client: 설정을 가져와서 사용하는 애플리케이션
2. Spring Cloud Config Server 설정
Gradle 의존성 추가
ext {
set('springCloudVersion', "2024.0.0")
}
dependencies {
implementation 'org.springframework.cloud:spring-cloud-config-server'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
testRuntimeOnly 'org.junit.platform:junit-platform-launcher'
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
Spring Boot 애플리케이션 클래스
@EnableConfigServer
@SpringBootApplication
public class CloudConfigApplication {
public static void main(String[] args) {
SpringApplication.run(CloudConfigApplication.class, args);
}
}
application.yml (Config Server)
server:
port: 8888 # Config Server 실행 포트
management:
endpoints:
web:
exposure:
include: "*"
endpoint:
shutdown:
enabled: true
spring:
cloud:
config:
server:
git:
default-label: main # Git 브랜치 지정
uri: git@github.com:Ahngyuho/spring-cloud-config-test.git # Git 저장소 주소 (SSH 방식)
private-key: |
-----BEGIN OPENSSH PRIVATE KEY-----
# 개인키 삽입
-----END OPENSSH PRIVATE KEY-----
ignore-local-ssh-settings: true
strict-host-key-checking: false # 호스트 키 검증 해제 (테스트 환경에서만 사용)
3. 설정 테스트
git 에 api-product.yml 파일 생성
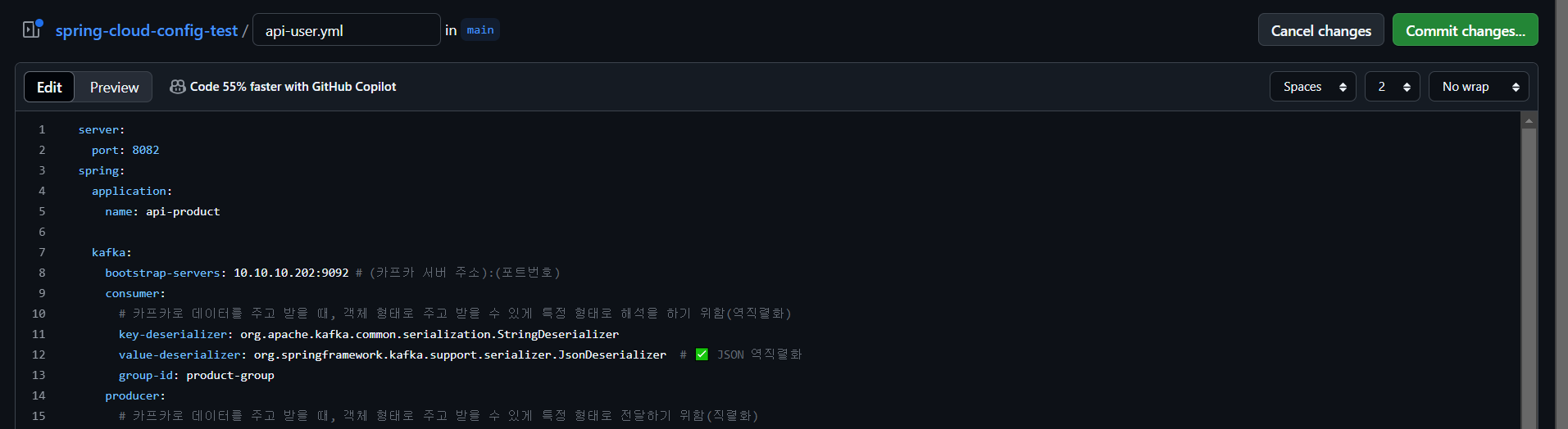
브라우저에 해당 url 입력
http://localhost:8888/api/product
결과
{
"name": "api",
"profiles": [
"product"
],
"label": null,
"version": "a45ded5d3ba7e057f604c6aa0e86d6f6a5fe8f96",
"state": "",
"propertySources": [
{
"name": "git@github.com:Ahngyuho/spring-cloud-config-test.git/api-product.yml",
"source": {
"server.port": 8082,
"spring.application.name": "api-product",
"spring.kafka.bootstrap-servers": "10.10.10.202:9092",
"spring.kafka.consumer.key-deserializer": "org.apache.kafka.common.serialization.StringDeserializer",
"spring.kafka.consumer.value-deserializer": "org.springframework.kafka.support.serializer.JsonDeserializer",
"spring.kafka.consumer.group-id": "product-group",
"spring.kafka.producer.key-serializer": "org.apache.kafka.common.serialization.StringSerializer",
"spring.kafka.producer.value-serializer": "org.springframework.kafka.support.serializer.JsonSerializer",
"spring.datasource.url": "jdbc:mariadb://192.0.3.30:3306/msa_shop",
"spring.datasource.driver-class-name": "org.mariadb.jdbc.Driver",
"spring.datasource.username": "agh",
"spring.datasource.password": "qwer1234",
"spring.jpa.database-platform": "org.hibernate.dialect.MariaDBDialect",
"spring.jpa.hibernate.ddl-auto": "update",
"spring.jpa.properties.hibernate.format_sql": true,
"logging.level.org.hibernate.SQL": "debug",
"logging.level.org.hibernate.orm.jdbc.bind": "trace"
}
}
]
}
4. Spring Cloud Config Client 설정
Gradle 의존성 추가
ext {
set('springCloudVersion', "2024.0.0")
}
dependencies {
implementation 'org.springframework.cloud:spring-cloud-starter-config'
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
application.yml (Config Client)
spring:
application:
name: api-product
config:
import: optional:configserver:http://localhost:8888 # config-server 의 도메인 주소
5. Spring Cloud Config 동작 방식
- Config Server 실행 (localhost:8888)
- Client가 spring.application.name을 기반으로 설정 요청
- Config Server가 Git에서 설정을 읽고 JSON 형태로 반환
- Client가 설정을 적용하여 애플리케이션 실행
6. Spring Cloud Config 최종 정리
Config Server 실행 | @EnableConfigServer 사용 |
Config Server가 설정 저장소로 Git 사용 | spring.cloud.config.server.git.uri 설정 |
Config Client에서 설정 가져오기 | spring.config.import: configserver:http://localhost:8888 |
SSH 키를 통한 Private Git Repo 접근 | private-key 설정 추가 |
설정이 정상적으로 불러와졌는지 확인 | curl http://localhost:8888/api-product/default |
'Server > Spring' 카테고리의 다른 글
[JPA] 영속성 컨텍스트 (0) | 2025.02.24 |
---|---|
@Transactional 에 대하여 (0) | 2025.02.24 |
HttpMessageConversionException 발생 원인과 해결책 알아보기 (0) | 2023.08.12 |